Old simple Palindrome using C#
This is just a code snippet for creating a palindrome program. Please find code below.
Project Structure
This is a console application built using VS 2012.
IDataType Interface
namespace CodeSnippets.Palindrome { public interface IDataType<T> { bool IsPalindrome(T data); } }
Integer Palindrome
Implements IDataType interface.
namespace CodeSnippets.Palindrome { public class IntegerPalindrome : IDataType<int> { public bool IsPalindrome(int data) { bool isValid = false; int palindrome = data; int reverse = 0; while (palindrome != 0) { int remainder = palindrome % 10; reverse = reverse * 10 + remainder; palindrome = palindrome / 10; } if (data == reverse) { isValid = true; } return isValid; } } }
String Palindrome
Implements IDataType interface
using System; namespace CodeSnippets.Palindrome { public class StringPalindrome : IDataType<String> { public bool IsPalindrome(string data) { bool isValid = true; if (data != null && data.Length > 0) { int iterationLength = Math.Abs(data.Length) / 2; string lowerData = data.ToLower(); for (int j = 0; j < iterationLength; j++) { if (lowerData.Substring(j, 1) != lowerData.Substring(lowerData.Length - 1 - j, 1)) { isValid = false; break; } } } return isValid; } } }
PalindromeFactory class
Returns right type of object depending on data type.Example StringPalindrome if string is passed to test for palindrome. It implements only two data types String and Int in this code. More types can be added here.
using System; namespace CodeSnippets.Palindrome { public static class PalindromeFactory<T> { public static IDataType<T> getInstance(T data) { if (data.GetType() == typeof(String)) { return (IDataType<T>)new StringPalindrome(); } if (data.GetType() == typeof(int)) { return (IDataType<T>)new IntegerPalindrome(); } throw new NotImplementedException("Data type is not implemented in factory"); } } }
Palindrome class
We create instance of this class for determining a palindrome.
namespace CodeSnippets.Palindrome { public class Palindrome<T> { public bool IsPalindrome(T data) { return PalindromeFactory<T>.getInstance(data).IsPalindrome(data); } } }
Program class
This is main console application class. Provides entry point for the application to start.
using System; using CodeSnippets.Palindrome; namespace CodeSnippets { class Program { static void Main(string[] args) { var pInt = new Palindrome<int>(); var pString = new Palindrome<String>(); Console.WriteLine("Is 121 a Palindrome : " + pInt.IsPalindrome(121)); Console.WriteLine("Is 234534 a Palindrome : " + pInt.IsPalindrome(234534)); Console.WriteLine("Is Bhawesh a Palindrome : " + pString.IsPalindrome("Bhawesh")); Console.WriteLine("Is Malayalam a Palindrome : " + pString.IsPalindrome("Malayalam")); Console.ReadLine(); } } }
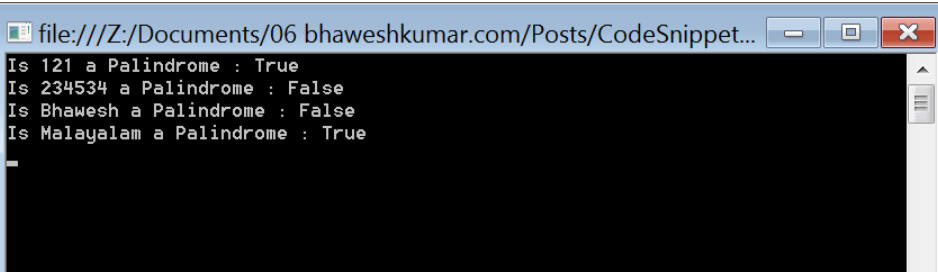
Palindrome output